This article focuses on the STM32 TMP36 Temperature Sensor and its integration with STM32 microcontrollers.
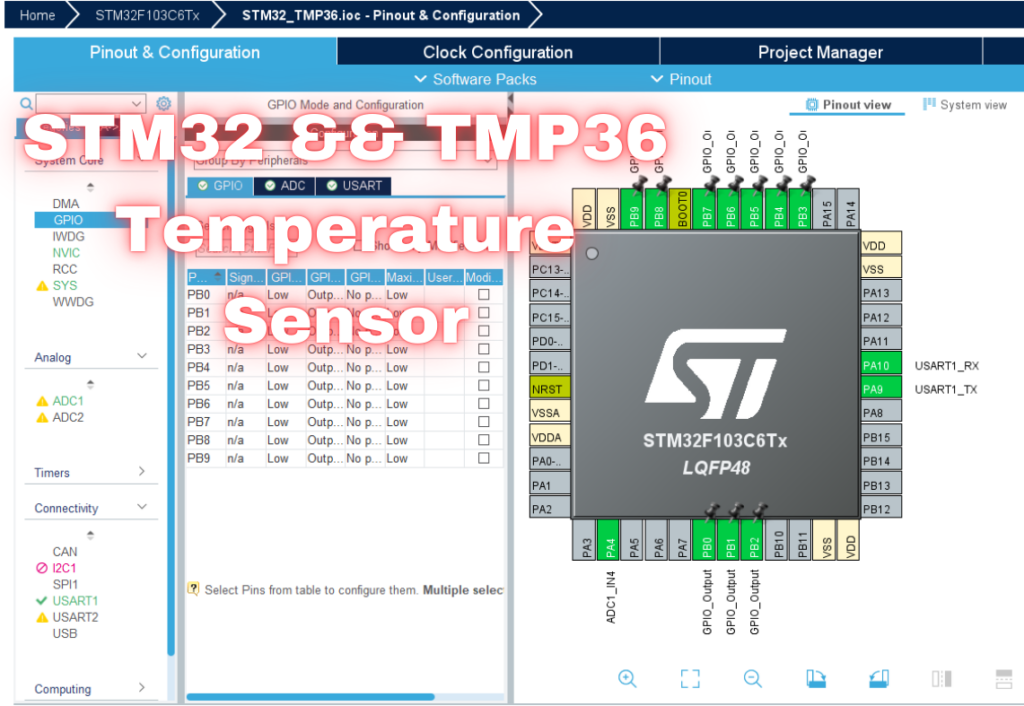
Things used in this project
Software apps and online services
1-Â STMicroelectronics STM32CubeMX
2-Â STMicroelectronics STM32CubeIDE
3-Â Proteus 8
Low-Level Integration of the TMP36 Temperature Sensor with STM32 Microcontrollers
In this project, we explore the integration of the TMP36 temperature sensor with ùùmicrocontrollers, emphasizing low-level interfacing techniques to maximize control and efficiency. By bypassing high-level libraries, we gain a deeper understanding of the sensor’s operation and enhance our ability to optimize performance for specific applications.
TMP36 Pinout and Basic Operation :
The TMP36 sensor is a low-voltage, precision centigrade temperature sensor. It operates at a supply voltage range of 2.7V to 5.5V, making it ideal for integration with STM32 microcontrollers. The sensor has three pins:
- VCC: Connects to the power supply (2.7V to 5.5V)
- GND: Ground
- VOUT: Analog voltage output that varies with temperature
The TMP36 provides an analog voltage output that is linearly proportional to the temperature in degrees Celsius. Specifically, the output voltage (in millivolts) can be calculated as:
Technical Specifications of TMP36Â :
- Operates from 2.7V to 5.5V
- Linear +10 mV/°C scale factor
- ±0.5°C linearity
- ±2°C accuracy over temperature (typical)
- Rated for full -40°C to 125°C range, operates up to +150°C
- Less than 50 μA current drain
- VOUT range: 0.1V at -40°C to 1.75V at 125°C
- VOUT at room temperature (25°C) is 0.75V
To kickstart this project, we’ll configure the STM32 microcontroller to interface with the TMP36 temperature sensor and display temperature readings on an LCD screen. Specifically, we’ll use the HAL library to initialize the ADC for reading the sensor output, and UART for debugging and monitoring purposes. We will also configure GPIO pins to control LEDs based on temperature thresholds. This setup will allow us to efficiently monitor temperature and provide visual alerts, thereby optimizing system responsiveness and user interaction.0
STM32CubeMX Configuration:
- Open CubeMX & Create New Project Choose The Target MCUÂ STM32F103C6Â & Double-Click Its Name
- Go To The Clock Configuration & Set The System Clock To 8MHz
Configuration for the GPIO Mode:
- Configure The GPIO Pins [PB0..PB9] as Output Pins
Configuration for the ADC
Modes:
- In the Categories tab, select the ADC1 & enable IN4
Configuration for the UART Mode:
- Enable USART1 Module (Asynchronous Mode)
- Set the USART1 communication parameters (baud rate =Â 115200, parity=NON, stop bits =1, and word length =Â 8bits)
- Generate The Initialization Code & Open The Project In CubeIDE
STM32CubeIDE Configuration:
- Write The Application Layer Code
- main.c
/* USER CODE END Header */ /* Includes ------------------------------------------------------------------*/ #include "main.h" /* Private includes ----------------------------------------------------------*/ /* USER CODE BEGIN Includes */ #include "LiquidCrystal.h" #include<string.h> #include<stdio.h> /* Private variables ---------------------------------------------------------*/ ADC_HandleTypeDef hadc1; UART_HandleTypeDef huart1; /* USER CODE BEGIN PV */ float Voltage_mV = 0; float Temperature_C = 0; float Temperature_F = 0; char msg[50]; /* USER CODE END PV */ int main(void) { /* Reset of all peripherals, Initializes the Flash interface and the Systick. */ HAL_Init(); /* Configure the system clock */ SystemClock_Config(); /* Initialize all configured peripherals */ MX_GPIO_Init(); MX_ADC1_Init(); MX_USART1_UART_Init(); /* USER CODE BEGIN 2 */ LiquidCrystal(GPIOB, GPIO_PIN_3, GPIO_PIN_4, GPIO_PIN_5, GPIO_PIN_6, GPIO_PIN_7, GPIO_PIN_8, GPIO_PIN_9); begin(16, 2); /* USER CODE END 2 */ /* Infinite loop */ /* USER CODE BEGIN WHILE */ while (1) { /* USER CODE END WHILE */ /* USER CODE BEGIN 3 */ HAL_ADC_Start(&hadc1); // Start ADC Conversion HAL_ADC_PollForConversion(&hadc1, 1); // Poll ADC1 and Timeout = 1mSec uint32_t adc_value = HAL_ADC_GetValue(&hadc1); // Read the ADC Conversion Result HAL_ADC_Stop(&hadc1); // Calculate voltage and temperature Voltage_mV = (((adc_value * 3.3) / 4095.0) - 0.5) * 1000; Temperature_C = Voltage_mV / 10; Temperature_F = (Temperature_C * 1.8) + 32; // Transmit temperature over UART char msg[100]; sprintf(msg, "TempC: %.2f C", Temperature_C); setCursor(0, 0); print(msg); sprintf(msg, "TempF: %.2f F", Temperature_F); setCursor(0, 1); print(msg); // LED Alerts for Temperature Levels if (Temperature_C > 60) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_1, GPIO_PIN_SET); // Red LED ON HAL_GPIO_WritePin(GPIOB, GPIO_PIN_2, GPIO_PIN_RESET); // Blue LED OFF HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_RESET); // Green LED OFF sprintf(msg, "LED: Red ON (Temperature > 60 degrees Celsius)\r\n"); HAL_UART_Transmit(&huart1, (uint8_t*)msg, strlen(msg), HAL_MAX_DELAY); } else if (Temperature_C < 20) { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_2, GPIO_PIN_SET); // Blue LED ON HAL_GPIO_WritePin(GPIOB, GPIO_PIN_1, GPIO_PIN_RESET); // Red LED OFF HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_RESET); // Green LED OFF sprintf(msg, "LED: Blue ON (Temperature < 20 degrees Celsius)\r\n"); HAL_UART_Transmit(&huart1, (uint8_t*)msg, strlen(msg), HAL_MAX_DELAY); } else { HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_SET); // Green LED ON HAL_GPIO_WritePin(GPIOB, GPIO_PIN_2, GPIO_PIN_RESET); // Blue LED OFF HAL_GPIO_WritePin(GPIOB, GPIO_PIN_1, GPIO_PIN_RESET); // Red LED OFF sprintf(msg, "LED: Green ON (20 degrees Celsius <= Temperature <= 60 degrees Celsius)\r\n"); HAL_UART_Transmit(&huart1, (uint8_t*)msg, strlen(msg), HAL_MAX_DELAY); } HAL_Delay(2000); clear(); } /* USER CODE END 3 */ }
Proteus Configuration :
- Open Proteus & Create New Project and click next
- Click on Pick Device
- Search for STM32F103C6 & LED-RED & LED-GREEN , LED_BLUE, TMP36
- Click on Virtual Instruments Mode then choose Terminal
- Click on Terminal Mode then choose (DEFAULT & POWER &GROUND)
- finally make the circuit below and start the simulation
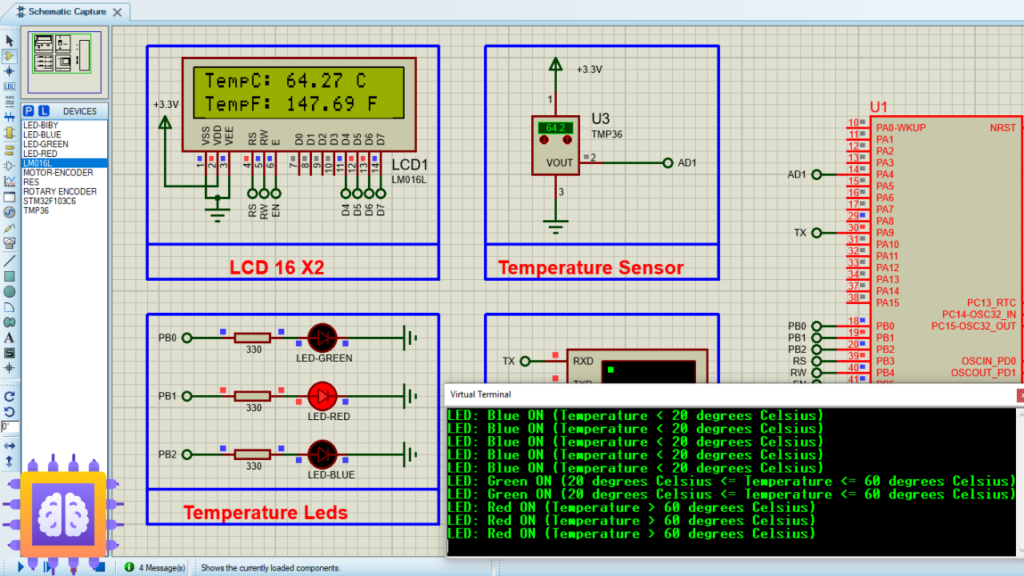
That’s all!
If you have any questions or suggestions don’t hesitate to leave a comment below
4 comments
very good! thanks so much
Thank you! I’m glad you found it helpful! 😊
how much baude rate did you put in Virtual terminal
I used a baud rate of 115200 in the virtual terminal