In this article, we will guide you through interfacing the I2C HYT/SHT21 sensors with the PIC16F877 microcontroller for accurate temperature and humidity readings.
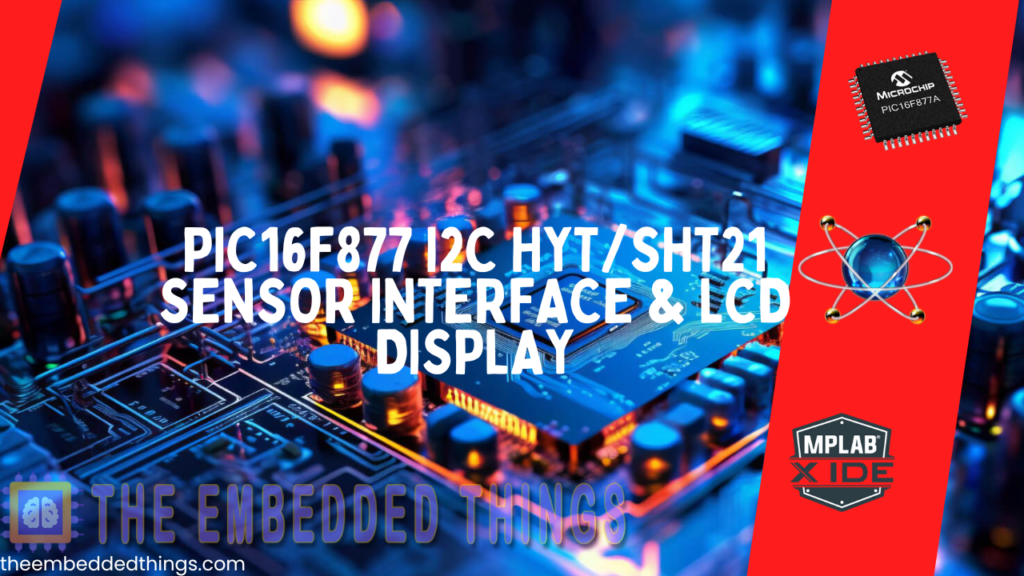
Interfacing HYT-271 and SHT21 I2C Sensors with PIC16F877A: A Comprehensive Guide to Temperature and Humidity Monitoring
In this project, we will explore how to interface the I2C HYT/SHT21 sensors with the PIC16F877A microcontroller. the I2C HYT/SHT21 sensors is a precise and reliable temperature and humidity sensor used in a variety of applications, including environmental monitoring and climate control systems.
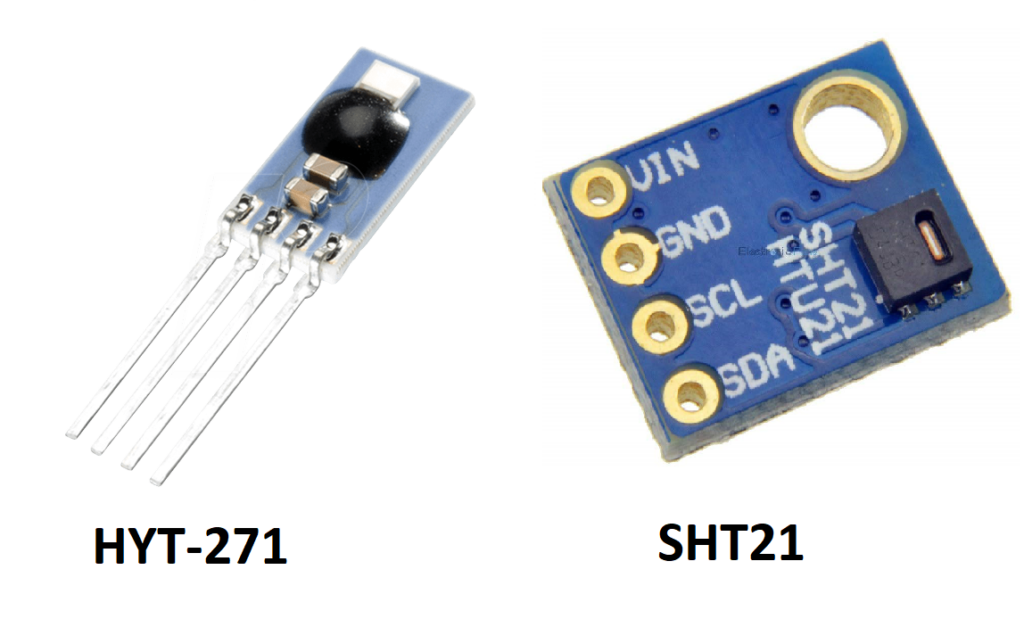
HYT-271 Temperature & humidity Sensor Overview
The HYT-271 is a small, durable digital humidity sensor that provides accurate measurements with an I²C interface. Offering ±1.8% RH and ±0.2°C accuracy, it is suitable for industrial devices, mass-market applications, and precise humidity transmitters. With its robust design, chemical resistance, and exceptional long-term stability, the HYT 271 is a reliable choice for monitoring environmental conditions without the need for frequent recalibration
Features:
- Measuring range: 0…100% RH, -40…125°C
- Accuracy: ±1.8% RH, ±0.2°C temperature
- Chemical and dew formation resistant
- Low hysteresis, compensated linearity error, and temperature drift
- Operating voltage: 2.7…5.5 V
- Nominal current consumption: 1 μA (25°C, sleep mode)
- High-quality ceramic substrate
- SIL connections, plug-in type, RM 1.27 mm
- RoHS compliant
- Dimensions: 10.2 x 10.5 x 5.1 mm
Accuracy and Repeatability Testing
The accuracy of the HYT 271 sensor is evaluated at 23°C and an operating voltage of 3.3 V, with measurements taken during an increase in humidity. This accuracy does not include the Tk-residual error, residual linearity error, or the effects of hysteresis.
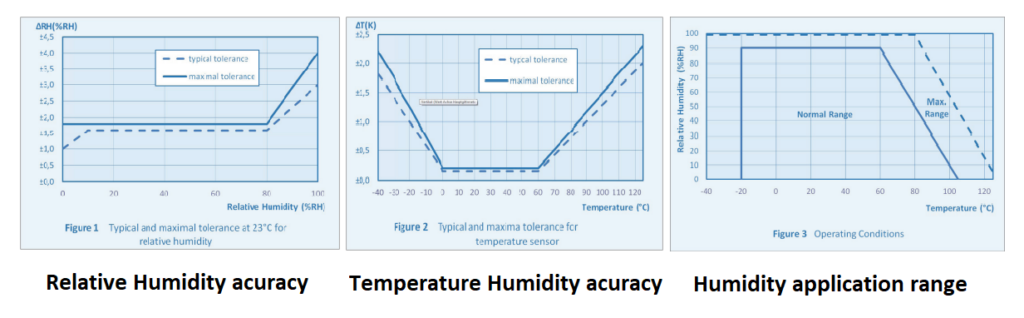
For repeatability testing, measurements are taken in the same direction of humidity change, without considering the hysteresis effect. Additionally, the sensor’s maximum dew point is limited to 80°C.
Applications:
- Handheld measurement instruments
- Humidity transmitters
- Industrial applications
- Sensor and measurement technology
SHT21 Humidity and Temperature Sensor Overview
The SHT21 from Sensirion is a compact and reliable humidity and temperature sensor, offering calibrated, linearized digital I²C signals. It integrates a capacitive humidity sensor, bandgap temperature sensor, and analog/digital circuitry within a small CMOSens® chip. With excellent accuracy, stability, and low power consumption, it’s ideal for various applications. Its small DFN package (3×3 mm) makes it suitable for space-constrained designs
Features
- Form Factor: Small DFN package (3 x 3 mm, 1.1 mm height)
- Sensor Type: Capacitive humidity sensor and bandgap temperature sensor on a CMOSens® chip
- Output: Digital I²C signal, calibrated and linearized
- Accuracy: Excellent sensor performance with minimal power consumption
- Customization: Resolution adjustable via command (8/12 bit to 12/14 bit for RH/T)
- Reliability: High long-term stability and proven reliability
- Testing: Each sensor is individually calibrated and tested, with lot identification and an electronic identification code
Accuracy and Specifications Summary
The SHT21 sensor provides a default 14-bit temperature and 12-bit humidity resolution, adjustable via user commands. Accuracy is tested at 25°C and 3.0 V, excluding hysteresis and drift. Its operating range is 0-80% RH, with minimal long-term drift (<0.5% RH/year). Power dissipation and supply current are based on a fixed 3.0 V supply, and response time is influenced by the sensor substrate’s heat conductivity.
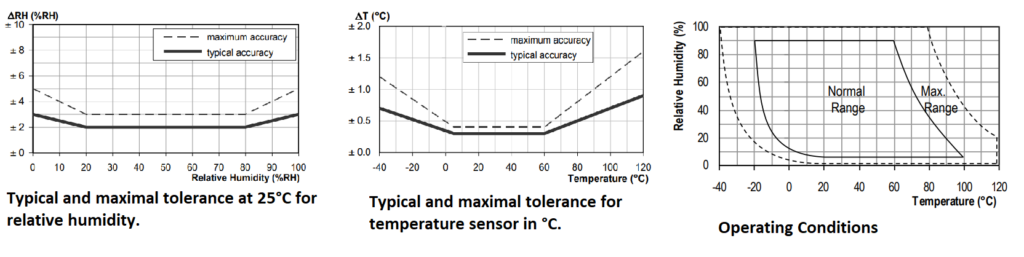
Interfacing HYT271 and SHT21 Humidity & Temperature Sensors with LCD Display using I2C on PIC
This project interfaces the HYT271 and SHT21 temperature and humidity sensors with a PIC microcontroller using I2C communication. It reads data from both sensors and displays the results on a 16×2 I2C LCD. The system continuously updates the display every second with temperature and humidity values. This demonstrates multi-sensor integration, I2C communication, and real-time data visualization on an embedded system.
HYT-271 Sensor Driver Header
This header file defines functions for interfacing the HYT-271 temperature and humidity sensor with a PIC microcontroller using I²C. It includes functions for initializing the sensor, starting measurements, and retrieving the measured temperature and humidity. You can find a detailed explanation of these functions in the video on YouTube .
#ifndef HYT_271_H #define HYT_271_H #include <xc.h> #include "i2c.h" // Sensor I2C Address #define SLAVE_ADDR 0x28 // Function Declarations void HYT271_Init(void); // Initialize the sensor void HYT271_StartMeasurement(void); // Start measurement on the sensor void HYT271_ReadData(void); // Read data from the sensor float HYT271_GetTemperature(void); // Get the measured temperature float HYT271_GetHumidity(void); // Get the measured humidity #endif // HYT_271_H
SHT21 Sensor Driver Header
This header file provides function prototypes and constants for interfacing with the SHT2x temperature and humidity sensor using I²C communication. It includes functions to retrieve humidity, temperature, and dew point values, along with the necessary constants for dew point calculation. You can find a detailed explanation and implementation of these functions in the video on YouTube for a step-by-step guide.
#ifndef SHT2X_H #define SHT2X_H #include <stdint.h> #include <math.h> #include "i2c.h" // Constants for dew point calculation #define WATER_VAPOR 17.62f #define BAROMETRIC_PRESSURE 243.5f // SHT2x sensor address and commands #define SHT2x_ADDRESS 0x40 #define TEMP_HOLD_CMD 0xE3 #define RH_HOLD_CMD 0xE5 #define TEMP_NOHOLD_CMD 0xF3 #define RH_NOHOLD_CMD 0xF5 // Function prototypes float SHT2x_GetHumidity(void); float SHT2x_GetTemperature(void); float SHT2x_GetDewPoint(void); #endif
HYT271 and SHT21 Sensor Data Display on LCD
The code initializes the sensors and LCD, retrieves temperature and humidity data from both the HYT271 and SHT21 sensors, and displays the values on the LCD screen. The first row of the display shows the data from the HYT271 sensor, while the second row displays the data from the SHT21 sensor. A 1-second delay is implemented between readings to ensure continuous updates on the display. For further details on the display library used in this project, you can find it here.
#include <xc.h> #include "I2C.h" #include "SHT2x.h" #include "HYT-271.h" #include "jhd_lcd.h" #include <stdio.h> // LCD Address #define LCD_ADDRESS 0x7C // Create a buffer for formatted display text char displayText[17]; void main(void) { // Initialize I2C and HYT271 sensor HYT271_Init(); // Initialize LCD LCD_Init(LCD_ADDRESS); LCD_Clear(); while(1) { // Start sensor measurement for HYT271 HYT271_StartMeasurement(); // Get the processed temperature and humidity values from HYT271 float temperatureHYT = HYT271_GetTemperature(); float humidityHYT = HYT271_GetHumidity(); // Read Temperature and Humidity from SHT21 sensor float SHT21_temperature = SHT2x_GetTemperature(); float SHT21_humidity = SHT2x_GetHumidity(); // Display the information from both sensors LCD_SetCursor(0, 0); // Set cursor to first row // Format the string for displaying on the LCD sprintf(displayText, "HYT:%.1fC %.1f%%", temperatureHYT, humidityHYT); LCD_Print_String(displayText); // Print the formatted string for HYT-271 // Display HTU21D data on the second row LCD_SetCursor(0, 1); // Set cursor to second row sprintf(displayText, "SHT:%.1fC %.1f%%", SHT21_temperature, SHT21_humidity); LCD_Print_String(displayText); // Print the formatted string for HTU-21D // Wait before next reading __delay_ms(1000); } }
Proteus Configuration :
- Open Proteus & Create New Project and click next
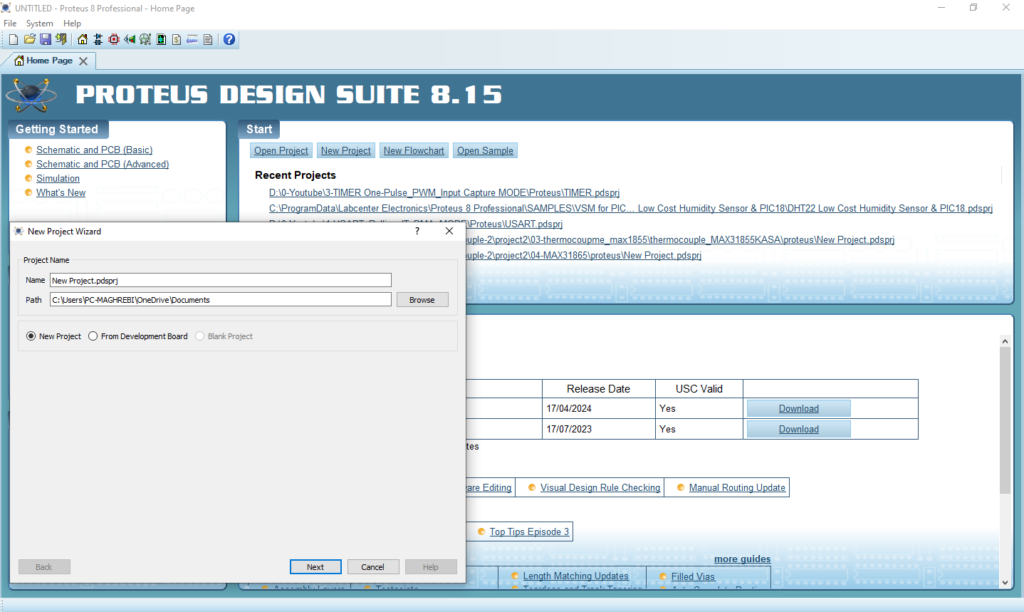
- Click on Pick Device
- Search for PIC16F877A & SHT21 & HYT-271& JHD-2X16-I2C
- Click on Virtual Instruments Mode then choose I2C DEBUGGER

- Click on Terminal Mode then choose (DEFAULT & POWER &GROUND)
- finally make the circuit below and start the simulation
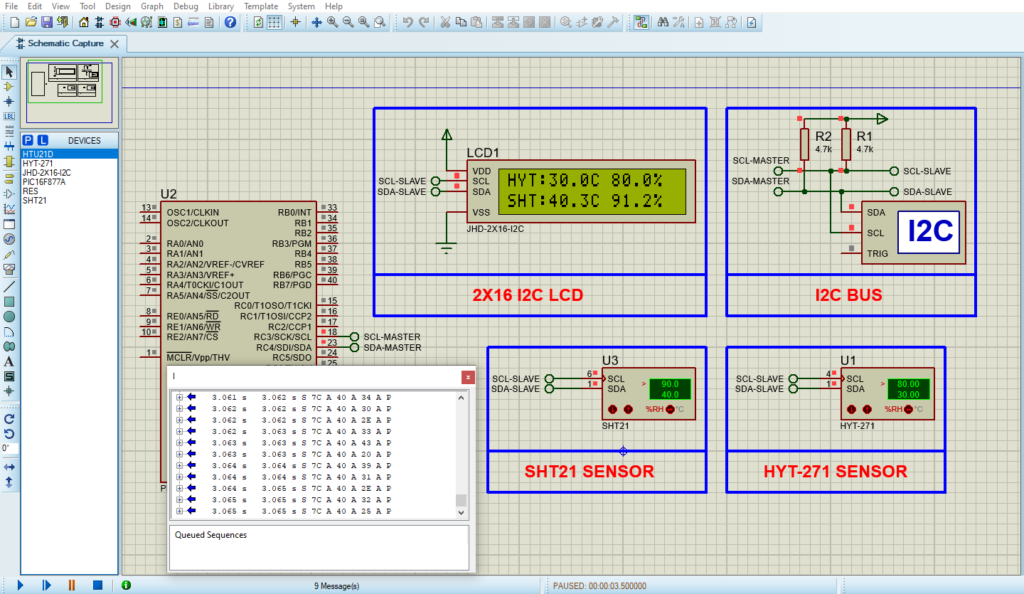
That’s all!
If you have any questions or suggestions don’t hesitate to leave a comment below
1 comment
[…] In this project, we will explore how to interface the TH02 I2C sensor with the PIC16F877A microcontroller for accurate temperature and humidity monitoring, making it ideal for embedded systems requiring precise environmental measurements. If you’re interested in similar projects, check out our “PIC16F877 I2C HYT/SHT21 Sensors Interface & I2C LCD Display.“ […]